Python theoretical interview Q&A:
- How many versions in Python? and what is the difference in between them?
- Describe variable? and how we create variables?
- What are Python identifiers? Is there any regulations to make identifiers?
- What do you mean by reserved keywords in Python?
- Do you know about indentation, and what's the purpose of it?
- How the comments in Python will help us? Multiline comments?
- Describe operators in Python?
- What is the difference between membership operators and identity operators?
- What are the control statements in Python? For what purpose they are useful?
- Describe if-elif-else?
- What do you mean by looping concept and explain different looping techniques?
- What is the difference between break & continue statements?
- Describe data structures in python? and it's types?
- What is the difference between list and tuple?
- Classify mutable and immutable data structures in python?
- Describe set and it's features?
- What is the difference between tuple and set?
- What do you mean by frozenset?
- Differentiate hard copy and shallow copy?
- What do you mean by dictionary in python and how it is different from other data structures?
- Describe the purpose of functions?
- Explain global variable and local variable?
- What is the difference between functions and anonymous function/ lambda?
- How the exception handling will help you in programming?
- What do you mean by module in python?
- What is the difference between module and library in python?
- Describe about inheritance and it's types?
- Define polymorphism? and discuss overriding and overloading?
- What do mean encapsulation?
- Describe data abstraction?
Answers and reference links:
1.How many versions in Python? and what is the difference in between them?For more information click here: Python 3 vs Python 2
2.Describe variable? and how we create variables?
A variable(string or character) is a reserved memory location, that stores a value or text. We use equal operator to assign a value, and we can perform numerical and string manipulations by using variable.
- Variable name can contain only letters, numbers, and underscore. ( A-Z, a-z, _ , 0-9 )
- Variable name can starts with letter or underscore
- Variable can't start with a number.
- Reserved keywords can't be used as variable
- Variable name can contain only letters, numbers, and underscore. ( A-Z, a-z, _ , 0-9 )
- Variable name can starts with letter or underscore
- Variable can't start with a number.
- Reserved keywords can't be used as variable.
- Single line comment: we use # symbol to write single line comments.
- Multiple line comment: we use docstrings( ''' ) to create multiple line comments.
- Arithmetic Operators
- Comparison or Relational Operators
- Assignment Operators
- Logical Operators
- Membership Operators
- Identity Operators
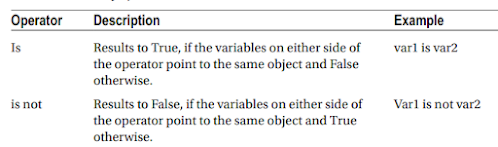
- Selection statements allow programmers to check a condition and based on the result will perform different actions. There are two versions of this useful construct: (1) if and (2) if…else.
- A loop control statement(iteration) enables us to execute a single or a set of programming statements multiple times until a given condition is satisfied. Python provides two essential looping statements: (1) for (2) while statement.
if 100 % 2 ==0: if 101 % 2 ==0:
return 'Even' return 'Even'
else: else:
return 'Odd' return 'Odd'
elif: Sometimes, we may have a case where we want one of many possible clauses to execute, In such scenarios we use elif . The elif statement means " else if " , that always follows an if or another elif statement.
Ex:
x=100
if x > 100:
print( " x is greater than 100 ")
elif x < 100:
print(" x is less than 100 ")
else:
print (" x is equal to 100 ")
11. What do you mean by looping concept and explain different looping techniques?Looping concept is try to execute the code multiple times, the loop will iterate as long as the condition is true. We have two types of looping techniques in Python:
1. for loop and
2. while loop
Ex: fruits=[ 'Mango' , 'Banana' , 'Grapes' , 'Apple']
Tuple:
Tuple is a immutable list, means if it once defined it cannot be modified. Items with in tuple also accessed by index as like list.
Ex:
days_of_week=('Monday', 'Tuesday' , 'Wednesday' , 'Thursday' , 'Friday' , 'Saturday' , 'Sunday')
Set:
Set is data structure that contains an unordered collection of unique and mutable objects. Set can perform mathematical operations like Union, Intersection, Difference etc..
Ex: A={1,5.2,4,3,6} or A= set(1,5,2,4,3,6)
Dictionary:
A dictionary holds key-value pairs, which are referred as items while other data types only contains elements.
Ex: Student_roll number = { 'Raj' : 1 , 'Ram' : 2 , 'Krishna' : 3 }
14. What is the difference between list and tuple?
- List is a mutable type of data structure, where as tuple is a immutable one.
- In list we can add, remove, and replace the values but none of these will perform by tuple.
- In list we use square brackets[ ] to create, and in tuple we use parenthesis ( ).
- Performance wise tuple is faster than list.
- We use parenthesis ( ) to create a tuple, but in set we use curly braces { } or set keyword to create sets.
- Tuple is a ordered collection of elements, where as set is a unordered collection of elements.
- Sets are mutable type of data structure and tuple is immutable type of data structure.
- Tuple allows duplicate elements, but set don't allow duplicate elements.
- To create tuple we use parenthesis () and in set we use curly braces {} or set() keyword
- Tuple is a ordered collection of elements, but set is an unordered collection of elements.
- Set is mutable where as tuple is immutable.
- Set don't allow duplicates but tuple allow duplicates that is repeated elements.
To create function, use the def keyword followed by the name of the function. By simply calling the name of the function(you defined) reuse for that particular task.
def function_name():
22. Explain global variable and local variable?
While creating identifiers like functions and classes we create variable, these variables are classified into two categories 1.Global variable and 2. Local variable.
Global variable: The variable which is created with in the program and it is applicable outside as well as within program
Local variable: The variable which is created in program and it is applicable within program itself.
23.What is the difference between functions and anonymous function/ lambda?
A function is block of code used to perform particular task. Function is defined with a name and we can use this function as many times we want by just calling function name.
Lambda is also a function without a defined name, hence we call it as anonymous function.
24. How the exception handling will help you in programming?
Any error that happens while a Python program is being executed that will interrupt the expected flow of the program is called as exception. Your program should be designed to handle both expected and unexpected errors
We can handle exceptions in our Python program using try, raise, except, and finally statements.
Try and except: try clause can be used to place any critical operation that can raise an exception in our program, and an exception clause should have the code that will handle a raised exception.
25. What do you mean by module in python?
Any text file having ".py " extension contains python code is called module. Generally module will contain different python objects like function, classes, variables etc,. We have in-built modules as well as we can create an user defined module with our own requirements. We can use the module whenever we required by simply importing it.
For example to perform any mathematical calculations we use math module(in-built)
import math as m
26. What is the difference between module and library in python?
Module:
Any text file having ".py " extension contains python code is called module. Generally module will contain different python objects like function, classes, variables etc,.
Library:
A library is a collection of related modules or packages.
27. Describe about inheritance and it's types?
Inheritance is inherit the properties of another class. Inheritance will help us code reusability, less code. Here we have two types of classes 1.Parent class and 2. Child class.
Types of inheritance:
Single inheritance: When child class derived from only one parent class
Multiple inheritance: When child class is inherited from multiple parent classes
Multilevel inheritance: In this we have one parent class and one child class and one grand child class.
Hierarchical inheritance: In this more than one child classes are inherit from one parent class.
Hybrid inheritance: It is the combination of all single, multiple, multilevel and hierarchical inheritances
28. Define polymorphism? and Discuss overriding and overloading?
The process of modifying or adding more features to already defined method is called Polymorphism.
Overloading: The process of using one same operator for different use
Ex: we use '+' operator for add numbers as well as to concatenate the strings.
Overriding: In this the method defined in parent class will reuse in child class (inheritance).
29. Describe Encapsulation?
Encapsulation is a mechanism of wrapping variables and methods together as a single unit.
The variables in the class will be hidden from other class and can only accessed by the methods of their current class.
Comments
Post a Comment